Thatch for Platforms enables partners to build an embedded health insurance experience into their applications. Using a combination of REST APIs and JavaScript components, you can deliver a complete health benefits solution for employers and their employees.
Thatch for Platforms provides five core capabilities:
- Authentication and authorization to securely connect with our APIs
- Plan retrieval and quote generation to run quotes and retrieve available plans for prospective employers
- Employer onboarding to onboard companies to Thatch directly in your product
- Employee management to enroll and invite employees
- Reporting through data export in common formats to track and understand activity on Thatch
This guide walks through implementing the first four features in your application. The full API documentation is available through Swagger.
Prerequisites
Thatch for Platforms is only available to specific partners. Get in touch with us at platforms@thatch.ai to set up an account.
All requests made to the Thatch API are authenticated and authorized using your API key. Once you have a Thatch account, you can generate an API key in the Thatch dashboard.
Thatch uses Bearer Token authentication in its APIs. In all API requests to Thatch, include this HTTP header:
Authentication: Bearer <sk_YOUR_API_KEY>
Retrieve plans and create a quote
The Thatch for Platforms APIs support quote generation for prospective employers, without the burden of onboarding them into Thatch first. Your application can do all this in four quick API calls.
Create a prospect.
Create a prospect—a potential employer on your platform—through the
POST /api/partners/v1/quoting/prospects
endpoint:curl https://partners.thatchcloud.com/api/partners/v1/quoting/prospects \ -H 'Content-Type: application/json' \ -H 'Authorization: Bearer <sk_YOUR_API_KEY>' \ -d '{ "name": "Acme Corp", "email": "prospect@acme.test", }'
The API responds to a successful request with JSON data that includes the generated prospect ID, required in the next step.
Add employees to the prospect.
To determine what plans each employee is eligible for and to generate accurate quotes, add employees to the prospect through the
POST /api/partners/v1/quoting/prospects/{prospect_id}/employee
endpoint:curl https://partners.thatchcloud.com/api/partners/v1/quoting/prospects/{prospect_id}/employees \ -H 'Content-Type: application/json' \ -H 'Authorization: Bearer <sk_YOUR_API_KEY>' \ -d '{ "name": "Beatriz Peel", "date_of_birth": "2002-11-14", "zip": "94103", "dependents": [ { "relationship": "spouse", "date_of_birth": "2001-04-22" } ] }'
The
POST /api/partners/v1/quoting/prospects/{prospect_id}/employees/bulk_create
endpoint supports bulk adding of employees.Retrieve available plans.
Next, retrieve a list of available insurance plans for your prospect's employees, including details such as pricing, coverage options, and plan terms. Do this through the
GET /api/partners/v1/plans
endpoint:curl https://partners.thatchcloud.com/api/partners/v1/plans?year=2025&zip=94103\ &ages[]=22&ages[]=23&relationships[]=self&relationships[]=spouse&page[number]=1&page[size]=20 \ -H 'Authorization: Bearer <sk_YOUR_API_KEY>'
The API responds to a successful request with JSON data that includes the available plans for the employee:
"data": [ { "id": "mdpln_01j2w394ngkjh3dfqdqzac4ev4", "carrier_name": "Anthem", "friendly_name": "Silver 70 HMO 2850/50", "plan_type": "hmo", "metal_tier": "silver", ...
Generate a quote.
Finally, partners can generate quotes based on the selected plans and employer preferences, returning all necessary pricing details.
Create a quote through the
POST /api/partners/v1/quoting/prospects/{prospect_id}/quotes
endpoint:curl https://partners.thatchcloud.com/api/partners/v1/quoting/prospects/{prospect_id}/quotes \ -H 'Content-Type: application/json' \ -H 'Authorization: Bearer <sk_YOUR_API_KEY>' \ -d '{ "year": 2025, "benchmark": "score_tier_0", "employee_multiplier": 0, "first_dependent_multiplier": 0, "additional_dependent_multiplier": 0 }'
Create the employer
Once an employer is ready to commit to your platform with Thatch health benefits, create the employer profile in Thatch. Provide all the required information, collected by your platform, through the POST /api/partners/v1/employers
endpoint:
curl https://partners.thatchcloud.com/api/partners/v1/employers \
-H 'Content-Type: application/json' \
-H 'Authorization: Bearer <sk_YOUR_API_KEY>' \
-d '{
"email": "admin@acme.test",
"name": "Acme Corp",
"business_type": "c_corp",
"ein": "12-3456789",
"dba": "string",
"address_line1": "123 Main St",
"address_line2": "",
"city": "San Francisco",
"state": "CA",
"zip": "94108",
"phone_number": "415-555-1212"
}'
The API responds to a successful request with JSON data that includes the generated employer ID, used in the employer onboarding flow.
Implement the employer onboarding flow
Almost all of the employer onboarding work is handled by Thatch, using an embeddable iframe for seamless integration into your product. Simply create an onboarding session, embed the iframe, and handle the onboarding completion event.
Create a secure session.
Create a secure session through the
POST /api/partners/v1/employer_onboarding_sessions
endpoint:curl https://partners.thatchcloud.com/api/partners/v1/quoting/prospects \ -H 'Content-Type: application/json' \ -H 'Authorization: <sk_YOUR_API_KEY>' \ -d '{ "employer": "empl_01j2j3smtwx656y2tbqm7ty6gr" }'
The API responds to a successful request with JSON data that includes a
claim_url
, to be provided to the iframe.Embed the onboarding iframe in your product.
<iframe id="thatch-employer-onboarding" title="Thatch employer onboarding" src="<%= claim_url %>" />
The iframe handles all of the onboarding logic and data collection.
Handle the completion event.
When the employer finishes onboarding, the iframe sends an event to the parent window. You can catch this event and handle it as needed.
window.addEventListener('message', handleMessage, false); function handleMessage(event) { var eventData = JSON.parse(event.data); switch(eventData.message_type) { case 'thatch.employer_onboarding_complete': // Handle completion (perform backend request, redirect) break; } }
At this point, the employer also receives an email inviting them to log into their Thatch dashboard.
Enroll employees
With the employer onboarded, the final step is to add and enroll employees. The Thatch for Platforms APIs support employee management including:
- Adding employees
- Retrieving lists of employees associated with an employer
- Viewing an employee's current enrollment status and enrolled plans
Add employees.
Add employees to Thatch through the
POST /api/partners/v1/employees
endpoint:curl https://partners.thatchcloud.com/api/partners/v1/employees \ -H 'Content-Type: application/json' \ -H 'Authorization: Bearer <sk_YOUR_API_KEY>' \ -d '{ "employer_id": "empl_01j2j3smtwx656y2tbqm7ty6gr", "personal_email": "beatriz.peel@gmail.test", "work_email": "bpeel@acme.test", "first_name": "Beatriz", "last_name": "Peel" }'
This can be done through the API either before or after the employer onboards with Thatch. This can also be automated through Thatch's integrations with a variety of payroll service providers.
Invite employees to enroll.
Employers can invite employees to enroll in health benefits through the employer's Thatch dashboard.
Get support
If you have any questions or need help with your integration, reach out to us at platforms@thatch.ai to talk with a partner engineer.
Calculate insurance costs instantly →
Chat with us to get started
Our team of engineers and benefits experts are standing by.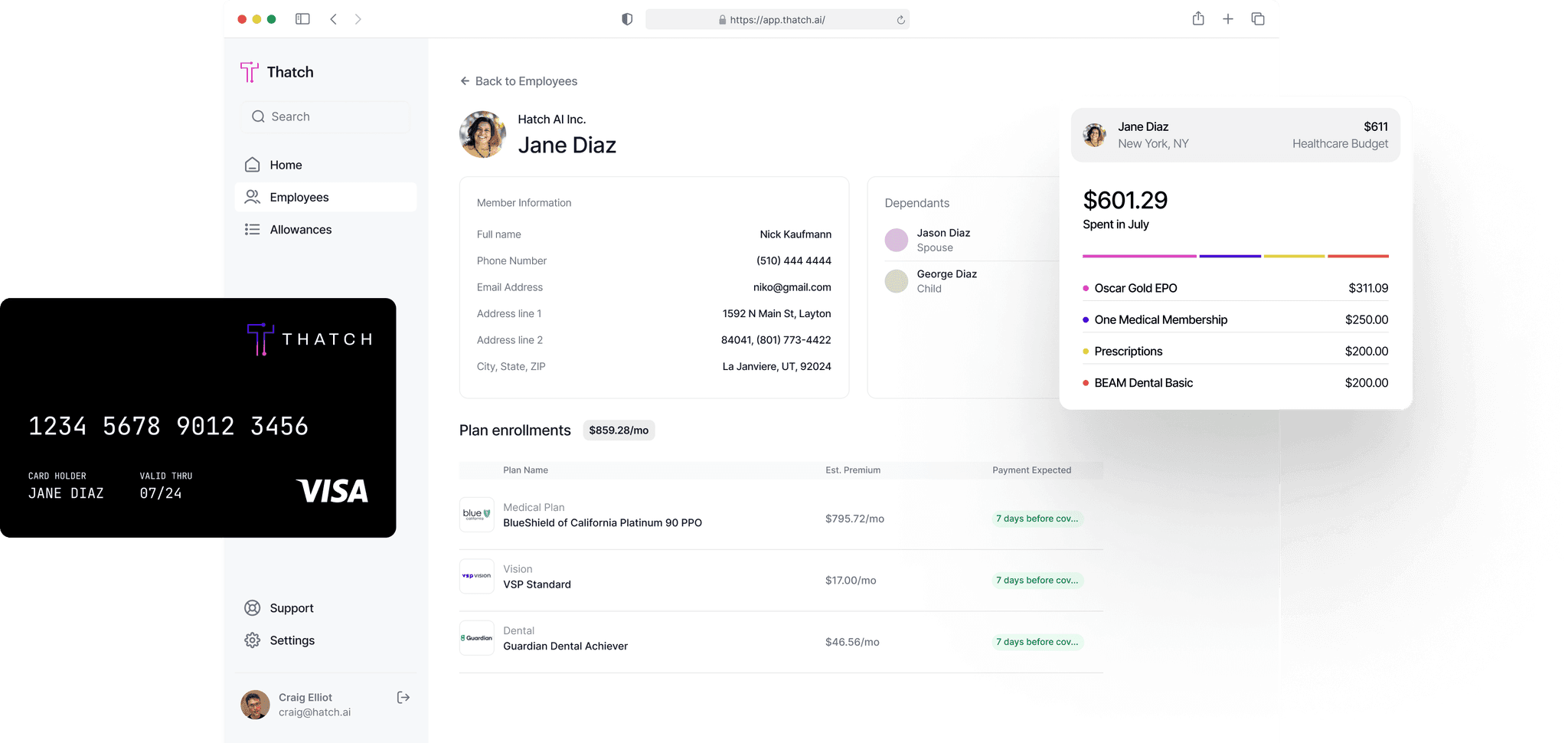